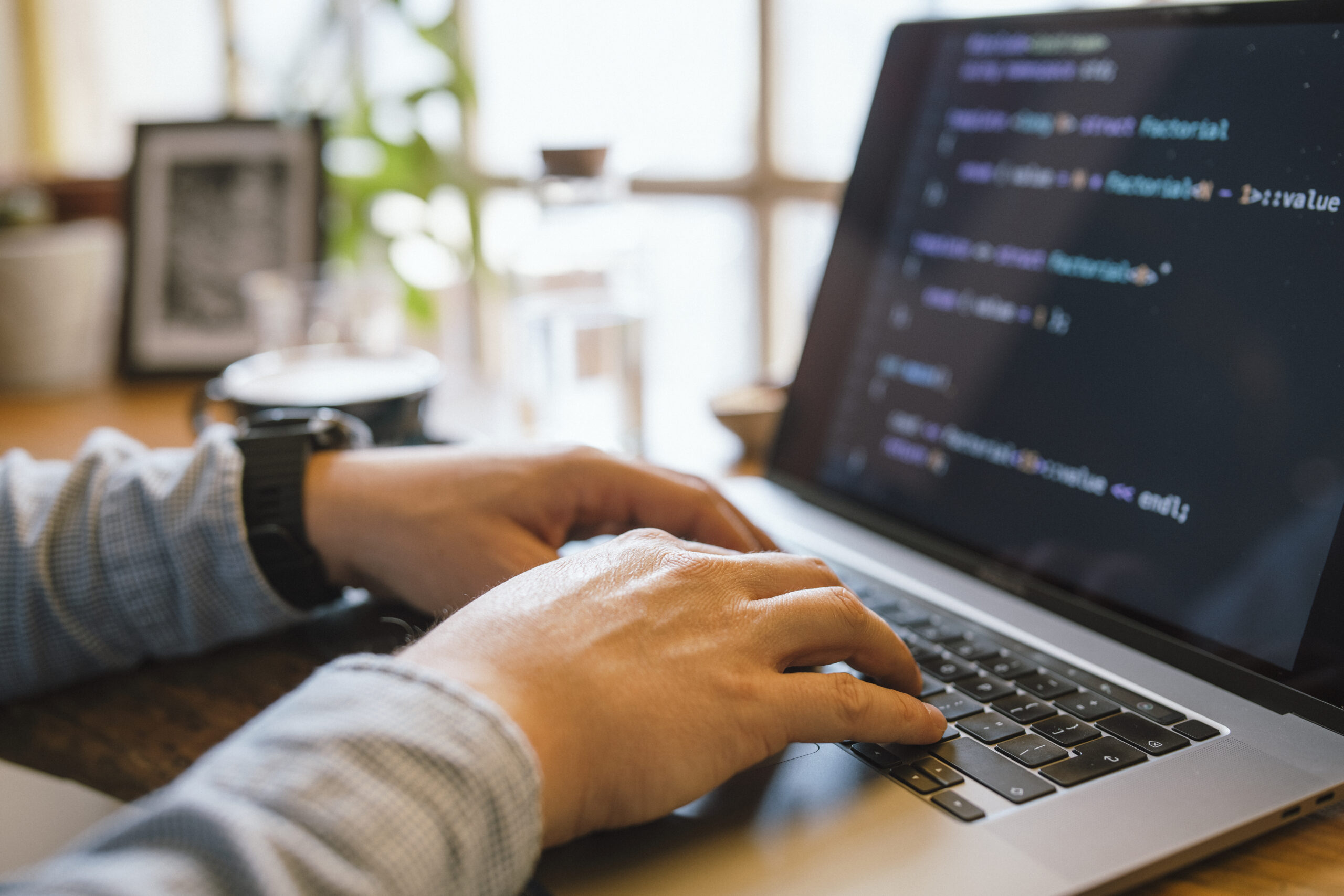
Debugging is Probably the most important — still normally overlooked — expertise within a developer’s toolkit. It's actually not almost correcting damaged code; it’s about being familiar with how and why things go wrong, and Understanding to Feel methodically to resolve difficulties proficiently. No matter whether you are a novice or possibly a seasoned developer, sharpening your debugging capabilities can preserve hrs of disappointment and drastically boost your productivity. Listed here are a number of methods to help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Tools
One of the fastest approaches developers can elevate their debugging skills is by mastering the equipment they use every single day. Even though composing code is one particular Section of advancement, realizing the best way to interact with it correctly through execution is Similarly essential. Modern development environments appear Outfitted with potent debugging abilities — but quite a few developers only scratch the surface of what these applications can do.
Take, such as, an Integrated Progress Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These resources allow you to established breakpoints, inspect the value of variables at runtime, action by means of code line by line, and even modify code to the fly. When utilized the right way, they Enable you to observe just how your code behaves throughout execution, and that is priceless for monitoring down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for front-conclude developers. They let you inspect the DOM, observe network requests, watch genuine-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can convert irritating UI troubles into workable tasks.
For backend or technique-amount developers, resources like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management around operating processes and memory administration. Discovering these tools could have a steeper Mastering curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into comfortable with version Handle methods like Git to grasp code heritage, obtain the exact moment bugs had been introduced, and isolate problematic adjustments.
In the long run, mastering your instruments usually means likely beyond default settings and shortcuts — it’s about developing an intimate knowledge of your improvement surroundings in order that when troubles occur, you’re not dropped at nighttime. The higher you recognize your instruments, the more time it is possible to commit solving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the situation
Among the most essential — and sometimes disregarded — actions in efficient debugging is reproducing the problem. Before leaping in the code or producing guesses, developers need to produce a regular surroundings or scenario where the bug reliably seems. With no reproducibility, fixing a bug results in being a video game of prospect, generally leading to squandered time and fragile code improvements.
Step one in reproducing an issue is accumulating as much context as possible. Talk to inquiries like: What actions triggered The problem? Which environment was it in — progress, staging, or creation? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it results in being to isolate the exact conditions beneath which the bug occurs.
When you finally’ve collected plenty of info, seek to recreate the trouble in your local environment. This might necessarily mean inputting precisely the same data, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, take into account writing automated assessments that replicate the sting circumstances or point out transitions involved. These exams don't just assist expose the situation but also avoid regressions Down the road.
Occasionally, The problem can be atmosphere-distinct — it'd happen only on specific operating techniques, browsers, or underneath individual configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t simply a move — it’s a mindset. It involves tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be now midway to correcting it. Which has a reproducible scenario, You should use your debugging resources a lot more efficiently, examination prospective fixes securely, and talk a lot more Obviously together with your group or consumers. It turns an abstract complaint right into a concrete obstacle — Which’s the place builders thrive.
Study and Comprehend the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes Erroneous. In lieu of observing them as aggravating interruptions, developers should master to treat error messages as immediate communications through the program. They frequently tell you what exactly occurred, where it transpired, and often even why it occurred — if you know how to interpret them.
Get started by looking through the message carefully As well as in total. Numerous builders, particularly when below time pressure, look at the very first line and immediately start out producing assumptions. But further while in the error stack or logs may well lie the correct root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and comprehend them initially.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line quantity? What module or purpose triggered it? These issues can manual your investigation and place you toward the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java frequently comply with predictable styles, and Understanding to acknowledge these can drastically accelerate your debugging course of action.
Some errors are obscure or generic, As well as in Those people circumstances, it’s important to look at the context in which the error transpired. Test related log entries, input values, and recent improvements during the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger sized problems and provide hints about likely bugs.
Finally, mistake messages are not your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, encouraging you pinpoint issues quicker, minimize debugging time, and become a far more successful and self-confident developer.
Use Logging Sensibly
Logging is One of the more powerful resources inside a developer’s debugging toolkit. When employed efficiently, it provides true-time insights into how an application behaves, assisting you comprehend what’s taking place under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts with knowing what to log and at what amount. Popular logging concentrations include things like DEBUG, Details, Alert, ERROR, and Deadly. Use DEBUG for thorough diagnostic details in the course of advancement, INFO for general situations (like prosperous start out-ups), WARN for possible issues that don’t crack the appliance, ERROR for actual complications, and Deadly once the system can’t proceed.
Steer clear of flooding your logs with too much or irrelevant facts. Excessive logging can obscure essential messages and decelerate your technique. Concentrate on key gatherings, state changes, enter/output values, and critical conclusion factors in your code.
Structure your log messages clearly and continuously. Contain context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting This system. They’re Specifically important in manufacturing environments the place stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about harmony and clarity. Which has a effectively-considered-out logging approach, it is possible to lessen the time it takes to spot difficulties, gain deeper visibility into your apps, and Increase the overall maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a complex endeavor—it's a form of investigation. To efficiently establish and fix bugs, developers need to technique the procedure similar to a detective resolving a thriller. This frame of mind can help stop working elaborate issues into manageable elements and comply with clues logically to uncover the basis bring about.
Get started by accumulating proof. Think about the symptoms of the issue: error messages, incorrect output, or efficiency concerns. Similar to a detective surveys a criminal offense scene, acquire as much appropriate data as you may without jumping to conclusions. Use logs, test cases, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Question oneself: What could possibly be leading to this actions? Have any variations a short while ago been designed to your codebase? Has this situation transpired prior to under identical situation? The purpose is usually to narrow down possibilities and detect probable culprits.
Then, examination your theories systematically. Attempt to recreate the problem inside of a managed surroundings. If you suspect a selected operate or component, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome lead you nearer to the truth.
Fork out close notice to modest particulars. Bugs generally conceal during the minimum envisioned locations—similar to a missing semicolon, an off-by-just one error, or maybe a race problem. Be complete and individual, resisting the urge to patch The difficulty without having fully comprehension it. Temporary fixes may possibly disguise the true challenge, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and discovered. Equally as detectives log their investigations, documenting your debugging system can conserve time for long run problems and support Many others realize your reasoning.
By imagining similar to a detective, developers can sharpen their analytical capabilities, tactic problems methodically, and grow to be more practical at uncovering concealed issues in sophisticated devices.
Generate Tests
Creating assessments is one of the best solutions to improve your debugging abilities and All round growth performance. Checks don't just help catch bugs early but additionally serve as a safety net that gives you self-assurance when generating improvements on your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint exactly where and when a problem takes place.
Get started with device assessments, which center on particular person capabilities or modules. These smaller, isolated assessments can speedily reveal no matter whether a particular piece of logic is Operating as expected. When a exam fails, you straight away know wherever to seem, drastically decreasing the time used debugging. Device exams are Particularly useful for catching regression bugs—issues that reappear just after Earlier getting set.
Next, combine integration exams and finish-to-end assessments into your workflow. These aid make sure that various aspects of your software function together efficiently. They’re specifically useful for catching bugs that come about in intricate methods with various elements or services interacting. If a thing breaks, your exams can show you which Section of the pipeline failed and beneath what circumstances.
Producing exams also forces you to definitely Believe critically regarding your code. To test a element effectively, you would like to grasp its inputs, expected outputs, and edge situations. This level of comprehension The natural way qualified prospects to raised code construction and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug may be a strong starting point. After the take a look at fails consistently, it is possible to deal with fixing the bug and look at your exam pass when The problem is solved. This approach makes sure that the exact same bug doesn’t return in the future.
In brief, composing checks turns debugging from the irritating guessing match right into a structured and predictable system—assisting you catch far more bugs, a lot quicker and more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to be immersed in the issue—looking at your display for hrs, hoping Option after solution. But Probably the most underrated debugging equipment is just stepping away. Using breaks aids you reset your brain, minimize disappointment, and sometimes see The problem from a new viewpoint.
When you're as well close to the code for Developers blog as well lengthy, cognitive fatigue sets in. You might start overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain turns into much less efficient at problem-resolving. A brief stroll, a coffee crack, or simply switching to a unique process for 10–15 minutes can refresh your aim. Quite a few developers report locating the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the qualifications.
Breaks also assist prevent burnout, Primarily all through more time debugging sessions. Sitting down in front of a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent permits you to return with renewed energy and also a clearer frame of mind. You would possibly abruptly notice a lacking semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, an excellent general guideline is usually to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver around, extend, or do something unrelated to code. It might feel counterintuitive, Specially less than restricted deadlines, but it really truly brings about quicker and simpler debugging in the long run.
In a nutshell, using breaks will not be a sign of weak point—it’s a wise strategy. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is an element of resolving it.
Discover From Just about every Bug
Every bug you experience is much more than simply A short lived setback—it's an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural problem, each can train you a thing important in the event you take some time to mirror and assess what went Erroneous.
Get started by inquiring yourself a couple of crucial inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved tactics like device tests, code assessments, or logging? The responses generally expose blind places with your workflow or knowledge and make it easier to Make more robust coding practices relocating forward.
Documenting bugs may also be a great behavior. Maintain a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or widespread problems—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends might be Specifically potent. Whether it’s via a Slack concept, a brief produce-up, or a quick knowledge-sharing session, serving to Other individuals avoid the similar concern boosts team performance and cultivates a more powerful Finding out culture.
Extra importantly, viewing bugs as lessons shifts your mindset from stress to curiosity. As opposed to dreading bugs, you’ll get started appreciating them as vital parts of your progress journey. In any case, a lot of the ideal builders usually are not those who compose fantastic code, but people who consistently find out from their issues.
Ultimately, Just about every bug you repair provides a completely new layer in your talent set. So up coming time you squash a bug, have a moment to reflect—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Strengthening your debugging competencies requires time, follow, and tolerance — however the payoff is big. It would make you a far more effective, assured, and able developer. Another time you're knee-deep within a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.